How to Unregister a Custom Post Type in WordPress
The function unregister_post_type
unregisters a custom post type in WordPress.
Preparation
Register the custom post type Book using the code below. Open the administration area and verify that it has a section to manage posts of the post type Book.
Translate source code strings for proper display in languages other than English.
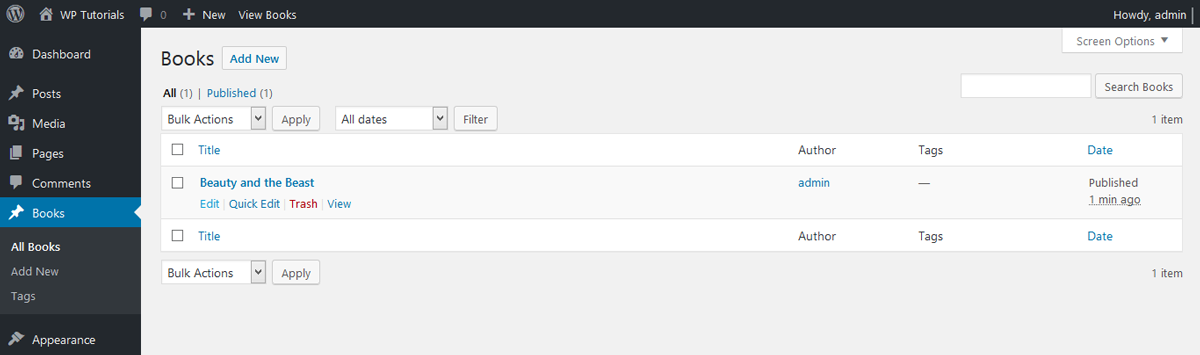
/*
* Registers the custom post type Book.
*/
function ns_register_book_cpt(){
// See all possible labels in the PHPDoc of the function get_post_type_labels
$labels = array(
'name' => __('Books', 'ns'),
'singular_name' => __('Book', 'ns'),
'menu_name' => __('Books', 'ns'),
'all_items' => __('All Books', 'ns'),
'add_new' => __('Add New', 'ns'),
'add_new_item' => __('Add New Book', 'ns'),
'edit_item' => __('Edit Book', 'ns'),
'new_item' => __('New Book', 'ns'),
'view_item' => __('View Book', 'ns'),
'view_items' => __('View Books', 'ns'),
'search_items' => __('Search Books', 'ns'),
'not_found' => __('No books found.', 'ns'),
'not_found_in_trash' => __('No books found in Trash.', 'ns'),
'archives' => __('Book Archives', 'ns'),
'filter_items_list' => __('Filter books list', 'ns'),
'items_list_navigation' => __('Books list navigation', 'ns'),
'items_list' => __('Books list', 'ns')
);
// See all possible attributes in the PHPDoc of the function register_post_type
$args = array(
'label' => __('Books', 'ns'),
'labels' => $labels,
'public' => true,
'exclude_from_search' => false,
'publicly_queryable' => true,
'show_ui' => true,
'show_in_nav_menus' => true,
'show_in_menu' => true,
'show_in_admin_bar' => true,
'hierarchical' => false,
'supports' => array('title', 'editor', 'author'),
'taxonomies' => array('post_tag'),
'has_archive' => true,
'rewrite' => array('slug' => 'books'),
'query_var' => 'book'
);
register_post_type('ns_book_cpt', $args);
}
add_action('init', 'ns_register_book_cpt', 10, 0);
Syntax
unregister_post_type($post_type)
unregisters the post type with the identifier $post_type
. It returns TRUE
on success, or WP_Error
if the post type does not exist or is a built-in post type.
Since post types are normally registered during the 'init'
action, call this function during the 'init'
action too, but late. Otherwise you could be trying to unregister a post type which has not been registered yet.
Example
Unregister the custom post type Book using the following code. It uses a big number at the third argument in the call to add_action
to try to place this task at the end of the 'init'
action.
/*
* Unregisters the custom post type Book.
*/
function ns_unregister_book_cpt(){
unregister_post_type('ns_book_cpt');
}
add_action('init', 'ns_unregister_book_cpt', 9999, 0);
Reload the administration area and verify that the Books menu has disappeared. WordPress has removed this type from the list of registered post types.
Data
Unregistering a post type does not delete posts of the post type from the database. It just tells WordPress that the post type no longer exists and WordPress removes all features created for the post type.
Remove the code that unregisters the Book post type, reload the administration area, and verify that the Books menu appears, including any posts you have created.
Use cases
You will need to unregister a post type rarely. A probable situation is when you use a theme or plugins that add more post types than needed. In this case, consider unregistering unused post types to keep the WordPress administration area clean.
Important: Unregister post types with caution and understand the consequences of each step. Otherwise you can conduct WordPress to a corrupt state.
Performance
Unregistering a post type adds more code to each page load. If performance is a concern, a better choice is to move unused post types to the bottom of the WordPress administration area.
Learn here how to reorder the menus of the administration area.
Further reading
I recommend the other tutorials in this series to learn more about post types in WordPress.
- Post Types in WordPress
- How to Register a Custom Post Type in WordPress
- How to Register a Custom Post Type Using a Plugin in WordPress
- How to Unregister a Custom Post Type in WordPress
- How to Modify a Post Type in WordPress
- How to Change the Slug of a Post Type in WordPress
- How to Check if a Post Type Exists in WordPress
- How to Get the Registered Post Types in WordPress
- How to Get the Attributes of a Post Type in WordPress
- How to Get the URL of a Post Type Archive in WordPress
- How to Add Custom Post Types to the Main Query in WordPress
Source code
The source code developed in this tutorial is available here.
Comments